Learn how Spring 4.2 simplifies handling transaction bound events (e.g. sent just after a database commit).
Introduction
As you probably already know (e.g. from my previous blog post) it is no longer needed to create a separate class implementing ApplicationListener
with onApplicationEvent
method to be able to react to application events (both from Spring Framework itself and our own domain events). Starting with Spring 4.2 the support for annotation-driven event listeners was added. It is enough to use @EventListener
at the method level which under-the-hood will automatically register corresponding ApplicationListener
:
@EventListener public void blogAdded(BlogAddedEvent blogAddedEvent) { externalNotificationSender.blogAdded(blogAddedEvent); }
Please notice that using domain objects in the events has notable drawbacks and is not the best idea in many situations. Pseudodomain objects in the code examples were used to not introduce unnecessary complexity.
Transaction bound events
Simple and compact. For “standard” events everything looks great but in some cases it is needed to perform some operations (usually asynchronous ones) just after the transaction has been committed (or rolled back). What’s then? Can the new mechanism be used as well?
Business requirements
First, a small digression – business requirements. Let’s imagine the super fancy blog aggregation service. An event is generated everytime the new blog is added. Subscribed users can receive an SMS or a push notification. The event could be published after the blog object is scheduled to be saved in a database. However, in in a case of commit/flush failure (database constraints violation, an issue with ID generator, etc.) the whole DB transaction would be rolled back. A lot of angry users with broken notification will appear at the door…
Technical issues
In modern approach to transaction management, transactions are configured declaratively (e.g. with @Transactional
annotation) and a commit is triggered at end of transactional scope (e.g. at the end of a method). In general this is very convenient and much less error prone (than the programmatic approach). On the other hand, commit (or rollback) is done automatically outside our code and we are not able to react in a “classical way” (i.e. publish event in the next line after transaction.commit()
is called).
Old school implementation
One of the possible solutions for Spring (and a very elegant one) was presented by indispensable Tomek Nurkiewicz. It uses TransactionSynchronizationManager
to register transaction synchronization for the current thread. For example:
@EventListener public void blogAddedTransactionalOldSchool(BlogAddedEvent blogAddedEvent) { //Note: *Old school* transaction handling before Spring 4.2 - broken in not transactional context TransactionSynchronizationManager.registerSynchronization( new TransactionSynchronizationAdapter() { @Override public void afterCommit() { internalSendBlogAddedNotification(blogAddedEvent); } }); }
The passed code is executed in the proper place in the Spring transaction workflow (for that case “just” after commit).
To provide support for execution in non-transactional context (e.g. in integration test cases which couldn’t care about transactions) it can be extended to the following form to not fail with java.lang.IllegalStateException: Transaction synchronization is not active
exception:
@EventListener public void blogAddedTransactionalOldSchool(final BlogAddedEvent blogAddedEvent) { //Note: *Old school* transaction handling before Spring 4.2 //"if" to not fail with "java.lang.IllegalStateException: Transaction synchronization is not active" if (TransactionSynchronizationManager.isActualTransactionActive()) { TransactionSynchronizationManager.registerSynchronization( new TransactionSynchronizationAdapter() { @Override public void afterCommit() { internalSendBlogAddedNotification(blogAddedEvent); } }); } else { log.warn("No active transaction found. Sending notification immediately."); externalNotificationSender.newBlogTransactionalOldSchool(blogAddedEvent); } }
With that change in a case of the lack of active transaction provided code is executed immediately. Works fine so far, but let’s try to achieve the same thing with annotation-driven event listeners in Spring 4.2.
Spring 4.2+ implementation
In addition to @EventListener
Spring 4.2 provides also one more annotation @TransactionalEventListener
.
@TransactionalEventListener public void blogAddedTransactional(BlogAddedEvent blogAddedEvent) { externalNotificationSender.newBlogTransactional(blogAddedEvent); }
The execution can be bound to standard transaction phases: before/after commit, after rollback or after completion (both commit or rollback). By default it processes an event only if it was published within the boundaries of a transaction. In other case the event is discarded.
To support the execution in non-transactional context the falbackExecution
flag can be used. If set to “true” the event is processed immediately if there is no transaction running.
@TransactionalEventListener(fallbackExecution = true) public void blogAddedTransactional(BlogAddedEvent blogAddedEvent) { externalNotificationSender.newBlogTransactional(blogAddedEvent); }
Summary
Introduced in Spring 4.2 annotation-driven event listeners continue a trend to reduce boilerplate code in Spring (Boot) based applications. No need to manually create ApplicationListener
implementations, no need to use directly TransactionSynchronizationManager
– just one annotation with proper configuration. The other side of the coin is that it is a little bit harder to find all event listeners, especially if there are dozens of them in our monolith application (though, it can be easily grouped). Of course, the new approach is only an option which could be useful in a given use-case or not. Nevertheless another piece of Spring (Boot) magic flood into our systems. But maybe resistance is futile?
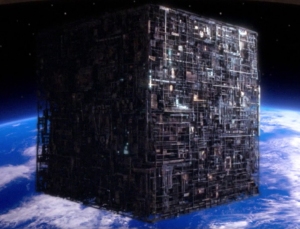
Resistance is futile?
Source: http://en.memory-alpha.wikia.com/wiki/Borg_cube
Please note that Spring Framework 4.2 is a default dependency of Spring Boot 1.3 (at the time of writing 1.3.0.M5 is available). Alternatively, it is possible to manually upgrade Spring Framework version in Gradle/Maven for Spring Boot 1.2.5 – it should work for most of the cases. Code examples are available from GitHub.
Btw, writing examples for that blog post gave me the first real ability to use the new test transaction management system introduced in Spring 4.1 (in the past I only mentioned it during my Spring training sessions). Probably, I will write more about it soon.
[…] >> Simpler handling of asynchronous transaction bound events in Spring 4.2+ [solidsoft] […]
[…] Simpler handling of asynchronous transaction bound events in Spring 4.2+ […]